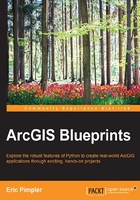
上QQ阅读APP看书,第一时间看更新
Reading data from the CSV file and writing to the feature class
The following steps will help you to read and write data from CSV file to a write to feature class:
- The main work of a tool is done inside the
execute()
method. This is where the geoprocessing of the tool takes place. Theexecute()
method, as shown in the following code, can accept a number of arguments, including the toolsself
,parameters
, andmessages
:def execute(self, parameters, messages): """The source code of the tool.""" return
- To access the parameter values that are passed into the tool, you can use the
valueAsText()
method. Add the following code to access the parameter values that will be passed into your tool. Remember from a previous step that the first parameter will contain a reference to a CSV file that will be imported and the second parameter is the output feature class where the data will be written:def execute(self, parameters, messages): inputCSV = parameters[0].valueAsText outFeatureClass = parameters[1].valueAsText schemaFeatureClass = parameters[2].valueAsText
- Import the
csv
andos
modules at the top of the script:import arcpy import csv import os
- Inside the
execute()
method, create thetry
/except
block that will wrap the code into an exception-handling structure:def execute(self, parameters, messages): """The source code of the tool.""" inputCSV = parameters[0].valueAsText outFeatureClass = parameters[1].valueAsText schemaFeatureClass = parameters[2].valueAsText try: except Exception as e: arcpy.AddMessage(e.message)
- Create the output feature class by passing in the
output
path,feature
class name, geometry type, schema feature class, and spatial reference:try: #create the feature class outCS = arcpy.SpatialReference(26910) arcpy.CreateFeatureclass_management(os.path.split(outFeatureClass)[0], os.path.split(outFeatureClass)[1], "point", schemaFeatureClass, spatial_reference=outCS)
- Create the
InsertCursor
object just below the line above where you created a new feature class:#create the insert cursor with arcpy.da.InsertCursor(outFeatureClass,("SHAPE@XY", "season", "date", "time", "hour", "temp", "bearing", "slope", "elevation")) as cursor:
- Open the
csv
file and read the data items that we need. There is a lot of data in thecsv
file, but we're not going to need everything. For our purposes, we are just going to pull out a handful of items, including the season, date, time, hour, coordinates, temperature, bearing, slope, and elevation. The following code block should be placed inside theWITH
statement. In this block of code, we open the file in read mode, create a CSV reader object, skip the header row, and then loop through all the records in the file and pull out the individual items that we need:with arcpy.da.InsertCursor(outFeatureClass,("SHAPE@XY", "season", "date", "time", "hour", "temp", "bearing", "slope", "elevation")) as cursor: #loop through the csv file and import the data into the feature class cntr = 1 with open(inputCSV, 'r') as f: reader = csv.reader(f) #skip the header next(reader, None) #loop through each of the rows and write to the feature class for row in reader: season = row[4] ## season dt = row[6] ## date tm = row[7] ## time hr = row[8] ## hour lng = row[10] lat = row[11] temperature = row[13] ## temperature bearing = row[17] ## bearing slope = row[23] ## slope elevation = row[28] ## elevation
- In the final code block for this tool, we'll add statements that convert the coordinates to a float datatype, encapsulate all the values within a Python list object, insert each row into the feature class, and update the progress dialog:
if lng != 'NA': lng = float(row[10]) lat = float(row[11]) row_value = [(lng,lat),season,dt,tm,hr,temperature,bearing,slope,elevation] cursor.insertRow(row_value) # Inserts the row into the feature class arcpy.AddMessage("Record number " + str(cntr) + " written to feature class") # Adds message to the progress dialog cntr = cntr + 1
- Check your code against the solution file found at
C:\ArcGIS_Blueprint_Python\solutions\ch2\ImportCollarData.py
to make sure you have coded everything correctly. - Double-click on the Import Collar Data tool to execute your work. Fill in the parameters, as shown in the following screenshot. The data we're importing from the
csv
file is from a single elk, which we'll callBetsy
: - Click on OK to execute the tool, and if everything has gone correctly, your progress dialog should start getting updated as the data is read from the file and written to the feature class.
- Close the progress dialog and open ArcMap with an empty map document file.
- In ArcMap, click on the Add Data from the drop-down list and select Add Basemap. Select the Topographic basemap and click on the Add button.
- Click on the Add Data button again, and navigate to the
Betsy
feature class that you created using the tool that you just created. Add this feature class to ArcMap. The data should be displayed in a cluster north-west of San Francisco in the Point Reyes National Seashore area. You will need to zoom in on this area. You should see something similar to what is shown in the following screenshot: - Save the map document as
C:\ArcGIS_Blueprint_Python\ch2\ElkMigration.mxd
. - In the next section, we'll make this data time-enabled so that we can get a better understanding of how this elk moves through its environment over time. Right now, the data just looks like one big cluster but, by making our data time-enabled, we'll be able to better understand the data.