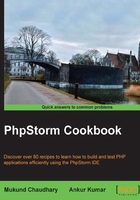
Creating a PHP project using Composer
Welcome to those who have taken the route to the right! Fasten your seatbelts, and get ready to create a new project in PHP.
Tip
Creating a new project in itself is a very mature decision, so you should always decide maturely and strategically. There might be situations in which, out of sheer enthusiasm, you opted to create a new project, and within a few days, you realize that much of the functionality you are planning to create has already been developed. You will end up doing a lot of copy-pasting work.
Getting ready
The principles of software engineering always teach students that a design should be high on coupling and low on cohesion. This means that an application program should use other programs but not depend on it. Composer in PHP is a system that helps you to adopt this methodology. Composer is a dependency manager for PHP, which allows other packages written in PHP to be included in other projects.
PhpStorm enables you to create projects that adhere to this principle. To create a new Composer project, you need to select the Composer Project option in the dropdown provided from the File | New Project menu item. The next question asked of you is to select the path of composer.phar
—from the local disk or from the website: http://www.getcomposer.org
How to do it...
Don't scratch your head out of utter confusion—more knowledge surely means more confusion, but that never means you should target zero knowledge to have no confusion at all! An executable, composer.phar
, causes this magic of handling the packages to occur. You must be thinking what exactly is it inside Composer that makes it such a buzzword these days—everyone seems to be talking about it. Perform the following steps:
- Go to https://getcomposer.org/download/, and you will see that there is the following command:
curl -sS https://getcomposer.org/installer | php
- If you have already installed composer prior to coming to cook with PhpStorm, you should specify
/path/to/composer.phar
, the path to where composer has been installed. - You can then select from the list of available packages in the left-hand side panel. On the right, you can see the corresponding description of the package selected. You can select the version of the package to be installed, and then click on OK.
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
How it works...
The command is actually a concatenation of two commands that get the contents of the installer script and execute that via the PHP command line. If you happen to open the specified link inside a web browser, you will see the entire PHP code behind Composer. Are you able to breathe freely (pun intended)? Back to work, the intention of the second part of the command is to execute the PHP content via the PHP command line.
Backtracking to the original topic, you were trying to select the right type of composer.phar
. Have a look at the following screenshot:

PhpStorm takes care of the remaining tasks in installing your-selected-package.
The Composer system generates a json
file with the name composer.json
, which contains the details about the requirements. The following is the composer.json
file for a project that has been created from scratch:
{ "name": "2085020/api_pingdom", "description": "pingdom api for php", "require": { "guzzle/guzzle": "v2.7.2" }, "authors": [ { "name": "Emagister", "email": "jcruz@emagister.com" } ], "autoload": { "psr-0": { "Emagister":"src" } } }
It is quite apparent that the json file conveys a lot of information to the reader. "name":
is the name of the package downloaded. "description":
is the description of the package downloaded. If you have a good memory, you will remember that there was a description in the right-hand panel of the package selection window. This description is exactly the same. "require":
is the name of the packages that this downloaded package depends upon. The json file specifies the name of the project as the key and the corresponding version number as the value. "authors":
is the author of the downloaded package. It includes the name and e-mail address of the author. "autoload":
is the coding standard, any of PSR-0, PSR-1, PSR-2, PSR-3, PSR-4, and the namespace mapping to the actual directory. The hierarchy goes like this: the coding-standard contains the value of the namespace mapping. Namespace mapping means the name as the key and the directory (relative to the project root) as the value.
PhpStorm and the Composer combination work wonders. When you order PhpStorm to download and arrange the packages, it will automatically arrange for you the include paths for the projects. No more warnings or errors relating to missing files will present a hindrance to your work now. Consider the following sreenshot:

Suppose you have already added a few packages in your project and you now realize that you need to have more of the packages. Not to worry says PhpStorm, as it provides you an option to add more packages in the form of Add dependency. Doing this is extremely easy. In the open project, you need to go to Tools | Composer | Add dependency. There will be a familiar pop up that will ask you to select a package name and proceed to install. The remainder of the tasks will, as usual, be taken care of by PhpStorm. The result you will see will be in the form of some downloaded files and folders besides a change in your composer.json
file for this particular project. One such composer.json
file is as shown in the following code snippet:
{ "name": "redis/ranking", "description": "Wrapping Redis's sorted set APIs for specializing ranking operations.", "keywords": ["redis", "ranking", "sort", "realtime"], "homepage": "https://github.com/matsubo/redis-ranking", "type": "library", "license": "PHP3", "require" : { "php": ">=5.3.0", "lib/curl": "0.3", "php-curl-class/php-curl-class": "1.0.1", "php-mod/curl": "1.0", "development/curl": "dev-master", "phpdocumentor/phpdocumentor": "2.0.0b7", "abhi/tangocard": "dev-master", "10up/wp_mock": "dev-master" }, "authors": [ { "name": "Yuki Matsukura", "email": "matsubokkuri@gmail.com" } ], "require": { }, "require-dev": { "devster/ubench": "1.1.*-dev", "phpunit/phpunit": "3.*" }, "autoload": { "psr-0": {"Matsubo": "src/"} } }
The symbols have the usual meaning. The external dependencies have been added to the list according to the json
notation and are denoted by the single key required, where the value is the actual list of the dependencies having the key as their name and the corresponding versions as the value.