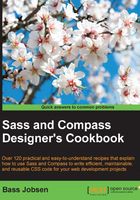
Using variables
When writing CSS code, you will have to repeat identical values and colors all around the place. Variables enable you to define commonly used values at a single place and solve the problem of repeating identical values in your code.
Getting ready
For this recipe, you will use the command-line Sass compiler and Compass. Read the Installing Sass for command line usage recipe of Chapter 1, Getting Started with Sass, to find out how to install it. In the Installing Compass recipe of Chapter 1, Getting Started with Sass, you can read about how to install Compass. You can edit the SCSS code in your favorite text editor. Finally, you will need a modern web browser to inspect the results.
How to do it...
The following steps help you to understand how to use variables in Sass:
- Create a file called
variables.scss
. This file should contain the following Sass code:@import 'compass/utilities/color/contrast'; // scss-lint:disable ColorKeyword $warning-color: orange; $warning-font-color: contrast-color($warning-color); .button-warning { background-color: $warning-color; border-color: darken($warning-color, 20%); border-radius: 20px; color: $warning-font-color; padding: 20px; &:hover { background-color: darken($warning-color, 30%); } }
- Compile the
variables.scss
Sass template from the previous step by running the following command in your console:sass --compass variables.scss variables.css
- Then, create a
index.html
HTML file that will link the compiledvariables.css
file and contain the HTML elements, as follows:<h1>Using variables in Sass</h1> <h1 class="warning">Using variables in Sass</h1> <button class="button-warning">Warning!</button>
- Open
index.html
in your web browser and find that it will look like that shown in the following image: - Now, you can change the value of the
@waring-color
variable in thevariables.scss
file fromorange
tored
and repeat the preceding steps.
How it works...
When you are creating the CSS code for you website or app, you may repeat some values more than once. You may possibly use a color pallet for your styling. When these commonly used values change, you should find and replace every usage in your CSS code. Variables enable you to define these values only once at a single place. Variables will make your code DRY (don't repeat yourself) and your projects more easy to maintain.
Variables begin with dollar signs, and are declared like CSS properties, using a colon between the name and the values and ending with a semicolon:
$variable: value;
Variable names can use hyphens and underscores interchangeably. Besides the data types supported by SassScript, any other valid CSS value can be assigned to a variable. SassScript supports seven data types: numbers
, quoted
and unquoted strings
, colors
, booleans
, nulls
, lists
, and maps
.
In Chapter 5, Built-in Functions, you can read about Sass built-in functions, which can be used to use and manipulate the data types as described earlier.
In this recipe, the built-in darken()
color function has been used. The darken()
function makes, as its name tells you already, a color darker. You can read more about functions to manipulate colors in the Color functions recipe of Chapter 5, Built-in Functions.
The contrast-color()
function used to set the value of the $warning-font-color
variable is a part of Compass. Compass is a style library for Sass. You can read more about Compass in Chapter 6, Using Compass, of this book. The contrast-color()
function will be described in a detailed way in the Creating color contrasts automatically recipe of Chapter 8, Advanced Sass Coding.
There's more...
When you start working with Sass variables, you will have to understand that these variables are scoped. Variables defined in the main scope, not nested inside a selector, mixin, or function, are available everywhere in your code. These kinds of variables are called global variables.
This can easily be seen when inspecting the following example SCSS code:
$color: red; .link { color: $color; }
The preceding code will compile into CSS as follows:
.link { color: red; }
On the other hand, variables defined inside a selector are called local variables. Local variables are only available inside their nesting (local scope). Consider the following SCSS code:
$primary: red; .link { $primary green; color: $primary; &:hover { color: $primary; } } p { color: $primary; }
The preceding Sass code will compile into CSS as follows:
.link { color: green; } .link:hover { color: green; }
p { color: red; }
Inside the .link
selector, the locally defined $primary
variable will be used.
In the Referencing parent selectors with & sign recipe of Chapter 4, Nested Selectors and Modular CSS, you can learn about the &
reference used to create the .link:hover
CSS selector. Now, you can see that the nested &:hover
used the $primary
variable defined in its parents' scope and not the globally defined $primary
color variable. The compiled CSS code of the p
selector shows that the global $primary
variable is still red
.
You can override variables in the same scope by defining a variable with the same name again. The new assigned value will only be used in the code after the overriding definition, which can be seen when inspecting the following example Sass code:
$primary: red; .link { color: $primary; } $primary: green; .other-link { color: $primary; }
The preceding SCSS code compiles into CSS code as follows:
.link { color: red; } .other-link { color: green; }
Finally, you can use the !global
keyword to override a globally defined variable in a local scope.
The following example demonstrates the effect of the !global
keyword in your code:
$color: red; .link { $primary: green !global; } .other-link { color: $primary; }
This compiles into the CSS code as follows:
.other-link { color: green; }
Here, the !global
keyword sets the value of the $primary
variable in the global scope to green.
When you use a variable before declaring it, the compiler will throw an Undefined variable
error and stop the compilation process. In the Declaring variables with !default recipe of this chapter, you can read how to prevent these errors by using the !default
keyword.
See also
When you study other CSS precompilers, such as Less or Stylus, you will find that the scope of definitions with the !global
keyword are a unique feature of Sass. You can compare different CSS precompilers and their features at http://csspre.com/compare/.