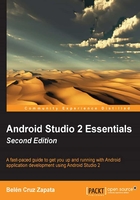
Creating a new project
To create a new project, click on the Start a new Android Studio project option from the welcome screen. If you are not in the welcome screen, then navigate to File | New Project. This opens the New Project wizard, as shown in the following screenshot:

Configuring the project
The fields that will be shown on the New Project wizard are as follows:
- Application name: This is the name shown in Google Play and the name that users see.
- Company Domain: This is the company or domain name that is used to create the package name of your application.
- Package name: This is the unique identifier of your application, usually in the
com.company_name.app_name
orreverse_company_domain.app_name
form. This form reduces the risk of name conflicts with other applications. A default package name is proposed, based on the Company Domain and Application name fields. You can change the package name by clicking on Edit. - Project location: This is the directory in your system in which the project is saved.
Complete the information for your project and click on the Next button. This will take you to the second screen. This screen allows you to select your platform and the minimum SDK your project will support on different devices.
Selecting the form factors
Because of the way Android has expanded to different types of devices, you may want to select one or more kinds of platforms and devices to be included in your project. For each type of device, you can select a different minimum SDK. The devices Android supports are as follows:
- Phone and Tablet: These are standard Android platforms used to create an application for phones and/or tablets.
- Wear: This is an Android Wear platform used to design applications for wearable devices such as smartwatches.
- TV: This is an Android TV platform used to design applications for big screens, such as those of television sets.
- Android Auto: This is an extension to the Android platform to enable your app to work in cars. There is no platform selector for Android Auto because it depends on a standard Phone and Tablet project. Your app needs to target Android 5.0 or higher to support Android Auto.
- Glass: This is an Android Glass platform used to design applications for Google Glass devices.
Once you've decided on your devices, you can choose the minimum SDK supported by your application. Devices with an older SDK will not be able to install your application. Try to reach a balance between supported devices and available features. If your application does not require a specific feature published in the newest SDKs, then you can select an older Application Programming Interface (API). The last dashboards published by Google about platform distribution show that 97.3 percent of the devices use Android 4.0.3 Ice Cream Sandwich or a later version. If you select Android 2.3.3 Gingerbread, then the percentage rises to almost 100 percent. You can check out these values by clicking on the Help me choose link. The official Android dashboards are also available at http://developer.android.com/about/dashboards/index.html.
To include any of the platforms and SDKs in your project, you need to have them installed in your system. The usual way to install a new platform is using a tool known as Android SDK Manager, which will be explained in Chapter 6, Tools. You don't have to use the manager now since the wizard to create a new project does all the work for you.
Check the Phone and Tablet option and select API 16
as the minimum SDK. After that, click on Next. The required components will be installed if necessary. If this is your case, click on Next again once the installation is completed. This will take you to the next screen where you can select the activity type.
Choosing the activity type
Activities are the components associated with the screens with which users interact in an application. The logic of an activity is implemented in a Java class with the name of the activity, which is created inside the source folder of your project. Android applications usually have multiple screens and are usually based on multiple activities. All the activities of an application have to be declared in the AndroidManifest.xml
file. It is mandatory in any Android application since it describes essential information about the application. In Chapter 3, Navigating a Project, you will learn about the project structure and the AndroidManifest.xml
file.
When an application is launched, it shows the main screen of the application. This step of the New Project wizard creates the main activity of your application, which is the main entry point of your application. You can indicate the type of activity you want to create as the main activity of your application. You can complete the creation of a new project without adding an activity, but you will need to add a main activity when you are finished creating your project. You can also change your main activity later in your project by modifying the AndroidManifest.xml
file. You will be able to add new activities to complete your application once your project is created. To create new activities you can use this same wizard step in the menu by navigating to File | New | Activity | Gallery.
Several types of activities that can be selected are as follows:
- Basic Activity: This template creates an activity with an action bar and a floating action button. The action bar includes a title and an options menu. Action bars can provide navigation modes and user actions. You can read more about action bars at http://developer.android.com/guide/topics/ui/actionbar.html.
Note
You can read more about floating action buttons at https://www.google.com/design/spec/components/buttons-floating-action-button.html.
The following screenshot shows Basic Activity:
If you select this template, Android Studio will create a project with two layout files (
activity_main.xml
andcontent_main.xml
) and the main activity class. The main layout (activity_main.xml
) declares the action bar and the floating action button, and includes the content layout (content_main.xml
) by adding the following XML declaration:<include layout="@layout/content_main" />
- Empty Activity: This creates a blank activity. Here is a screenshot showing Empty Activity:
- Fullscreen Activity: This template hides the system's user interface (such as the notification bar) in a fullscreen view. By default, the fullscreen mode is alternated with an action bar that shows up when the user touches the device screen. Fullscreen Activity is shown in the following screenshot:
If you select this template, Android Studio will create a project with a main activity and its main layout. The main activity contains all the logic to handle the fullscreen mode. For example, you will notice two helper methods that hide and show the action bar:
private void hide() { … } private void show() { … }
And you will notice the constant variables to configure whether the bar should hide automatically after a delay and the time of that delay:
/** * Whether or not the system UI should be auto-hidden after * {@link #AUTO_HIDE_DELAY_MILLIS} milliseconds. */ private static final boolean AUTO_HIDE = true; /** * If {@link #AUTO_HIDE} is set, the number of milliseconds to wait after * user interaction before hiding the system UI. */ private static final int AUTO_HIDE_DELAY_MILLIS = 3000;
- Google AdMob Ads Activity: This template creates an activity with a contained AdMob Ad. The purpose of this template is to display ads that allow monetizing your app. Here is a screenshot showing Google AdMob Ads Activity:
If you select this template, Android Studio will create a project with a main activity and its main layout. The main activity contains all the logic to create (
newInterstitialAd
method), load (loadInterstitial
method), and show (showInterstital
method) ads, which are saved in anInterstitialAd
object. - Google Maps Activity: This template creates a new activity with a Google map in a fragment. A fragment is a portion of user interface in an activity. Fragments can be reused in multiple activities, and multiple fragments can be combined in a single activity. See more about fragments at https://developer.android.com/guide/components/fragments.html. It is shown in the next screenshot:
If you select this template, Android Studio will create a project with a main activity and a layout containing the following map fragment:
<fragment xmlns:android="http://schemas.android.com/apk/res/android" xmlns:map="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/map" android:name="com.google.android.gms.maps.SupportMapFragment" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.mapstest.MapsActivity" />
- Login Activity: This template creates a view as a login screen, allowing the users to log in or register with e-mail and password.
If you select this template, Android Studio will create a project with a main activity and its layout. The main layout contains: a
ProgressBar
to show the login progress, anAutoCompleteTextView
for the user's e-mail, anEditText
for the user's password, and aButton
to sign in.The main activity includes code to autocomplete the e-mail, code to change the focus from one field to another, an
AsyncTask
class to perform the login in background, and code to show the progress of the login. - Master/Detail Flow: This template splits the screen into two sections: a left-hand-side menu and the details of the selected item on the right-hand side. On a smaller screen, just one section is displayed, but on a bigger screen, both sections are displayed at the same time.
If you select this template, Android Studio will create a project with two activities: the list activity and the detail activity. The project also contains the two layouts for both activities: the list layout and the detail layout. There are some additional classes, such as a detail fragment and layouts to create the master list.
- Navigation Drawer Activity: This template creates a new activity with a navigation drawer. It displays the main navigation options in a panel that is brought onto the screen from a left-hand-side panel. You can read more about navigation drawers at https://developer.android.com/design/patterns/navigation-drawer.html.
This template contains a main activity that implements the
NavigationView.OnNavigationItemSelectedListener
interface. This listener allows the activity to receive an event when the user selects an option from the drawer menu. Events are received in theonNavigationItemSelected
method implemented by the main activity:@Override public boolean onNavigationItemSelected(MenuItem item) { … }
The main layout is
DrawerLayout
, which contains aNavigationView
object. Extra layouts are also created in the project for the main content, the main top bar, and the drawer header views. - Scrolling Activity: This template creates an activity that scrolls vertically.
This template contains a main activity and a main layout. The main layout has a
Toolbar
and includes the scroll content layout:<include layout=""@layout/content_scrolling"" />
The content layout is
NestedScrollView
. - Settings Activity: This creates a preferences activity with a list of settings:
This template has two activities: the main activity and the preferences activity. The preferences activity extends the
PreferenceActivity
class and overrides the methods to build the preferences screen. The preferences content is configured using the XML files that define thePreferenceScreen
components. - Tabbed Activity: This creates a blank activity with an action bar similar to Basic Activity, but it also includes a navigational element. The navigational element can be a tabbed user interface (fixed or scrollable tabs), a horizontal swipe, or a spinner menu. The project content generated by Android Studio depends on the navigational element selected.
Select Empty Activity and click on Next.