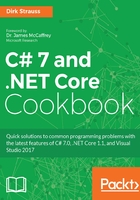
How it works...
In the code example, we set the student name and last name to specific values. This was just to illustrate the use of deconstruction. A more likely scenario would be to pass a student number to the Student class (in the constructor perhaps), as follows:
Student student = new Student(studentNumber);
The implementation within the Student class would then perform a database lookup using the student number passed through in the constructor. This will then return the student details. A more likely implementation of the Student class would probably look as follows:
public class Student
{
public Student(string studentNumber)
{
(Name, LastName) = GetStudentDetails(studentNumber);
}
public string Name { get; private set; }
public string LastName { get; private set; }
public List<int> CourseCodes { get; private set; }
public void Deconstruct(out string name, out string lastName)
{
name = Name;
lastName = LastName;
}
private (string name, string surname) GetStudentDetails(string studentNumber)
{
var detail = (n: "Dirk", s: "Strauss");
// Do something with student number to return the student details
return detail;
}
}
You will notice that the GetStudentDetails() method is just a dummy implementation. This is where the database lookup will start and the values will be returned from here. The code that calls the Student class now makes more sense. We call the Student class, pass it a student number, and deconstruct it to find the student's first name and surname.
Student student = new Student("S20323742");
var (FirstName, Surname) = student;
WriteLine($"The student name is {FirstName} {Surname}");