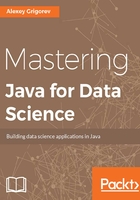
Commons IO
Like Apache Commons Lang extends the java.util standard package, Apache Commons IO extends java.io; it is a Java library of utilities to assist with I/O in Java, which, as we previously learned, can be quite verbose.
To include the library in your project, add the dependency snippet to the pom.xml file:
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.5</version>
</dependency>
We already learned about Files.lines from Java NIO. While it is handy, we do not always work with files, and sometimes need to get lines from some other InputStream, for example, a web page or a web socket.
For this purpose, Commons IO provides a very helpful utility class IOUtils. Using it, reading the entire input stream into string or a list of strings is quite easy:
try (InputStream is = new FileInputStream("data/text.txt")) {
String content = IOUtils.toString(is, StandardCharsets.UTF_8);
System.out.println(content);
}
try (InputStream is = new FileInputStream("data/text.txt")) {
List<String> lines = IOUtils.readLines(is, StandardCharsets.UTF_8);
System.out.println(lines);
}
Although we use FileInputStream objects in this example, it can be any other stream. The first method, IOUtils.toString, is particularly useful, and we will use it later for crawling web pages and processing the responses from web services.
There are a lot more useful methods for I/O in this library, and to get a good overview, you can consult the documentation available at https://commons.apache.org/io.