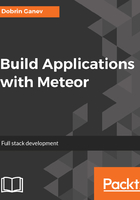
Improvements in the current code
In both the server and the client, we didn't provide an unsubscribing mechanism. For example, if you close the browser, the server will continue calling the newTime() function indefinitely.
Meteor.publish() provides some other useful APIs. When the client is subscribed for the first time, we can send a notification that the initial dataset was sent using this.ready(). This will call the onReady callback on the client.
We can also unsubscribe the client with this.stop() or listen whether the client subscription is stopped.
The publisher on the server:
Meteor.publish('time', function() {
let self = this;
self.added('time', id, time); // notify if record is added to the collection time
let interval = Meteor.setInterval(function() {
newTime();
}, 1000);
this.ready(); //
notify that the initial dataset was sent
self.onStop(function () {
self.stop()
console.log('stopped called')
Meteor.clearInterval(interval); // clear the interval if the the client unsubscribed
});
});
The subscriber on the client:
const handle = Meteor.subscribe("time", {
onReady: function() {
// fires when this.ready() called on the server.
}
});
handle.stop() // will call onStop(callback) on the server.
handle.ready() // returns true if the server called ready()
The clientTimer component:
Time = new Mongo.Collection('time');
const handle = Meteor.subscribe('time');
class Timer extends React.Component {
constructor(props) {
super(props);
}
shouldComponentUpdate() {
return this.props.handle.ready() && this.props.time.length > 0;
}
componentWillUnmount() {
this.props.handle.stop();
}
render() {
return <TimerDisplay time = { this.props.time }/>;
}
}
export default createContainer(() => {
return {
time: Time.find().fetch(), handle: handle
};
}, Timer);
Here, we passed the subscriber's handle as a prop to the component and in shouldComponentUpdate, we check whether the publisher sent the first dataset and whether it has any records. If yes, it will render the imerDisplay component.
When we unmount the component, we send a notification to the that we stopped the subscription.