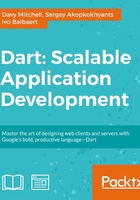
The Hello World server example
It is very simple to create a minimal Hello World server in Dart. Open the sample HelloWorld
file of this chapter in the Dart editor. This project is a command-line project, so the main.dart
file is placed in the bin
folder:
import 'dart:io'; void main() { HttpServer.bind('127.0.0.1', 8080).then( (server) { server.listen((HttpRequest request) { request.response ..write('Hello Dart World') ..close(); }); }); }
The output is shown in the following screenshot:

The dart:io
package is important for the server side. It allows us access to features that are not available to the code running in the web browser context due to security, such as filesystem and networking.
Launching the server program does not open up a web browser like in our previous application. You have to manually open a browser, then go to the address and to the port that you have bound to. Also, if a change is made in the server code, the application has to be stopped and restarted for the change to be seen
The HttpServer.bind
method takes two parameters—an IP address and a port number. The server is then attempted to be bounded to this network configuration, and if this is successful (and it usually only fails if the binding is already in use), the function is then executed.
The returned server object is set to listen to HTTP requests on that port. If any are received, the message Hello Dart World
is passed to the response
method of the request
object, and then, very importantly, the response is closed.
This simple example shows the structure of all HTTP-based server applications—bind to the network and then handle requests. Of course, handling requests and producing quality output is usually a good deal more complicated.
Note
If you are not too familiar with networking, here is a quick recap! IP (Internet Protocol) addresses help us identify machines (usually) on the network, and consist of 4 numbers that are separated by a full stop for example 192.168.0.1
.
Most computers have a special IP of 127.0.0.1
only for network communications on that machine. This is a computer's network address that is used to create a connection with itself and cannot be used by remote machines. Other than this distinction, it operates as an equal to any other IP address.
Ports help identify which application or service the connection is attached to. For an analogy, IPs are people's names and ports are the topics of conversation. To get the right information, you want to get both correct!
While the application is still running, go to main.dart
and change the message, that is the output, by adding !
to the end of the line. Refresh the browser page and you will find that nothing has changed. The Dart code runs on the server and does not get executed in the browser anymore, so the simple refresh feature to see changes is sadly gone now.
The good news is that starting and stopping a server application is very easy, and we can use any web browser to test the application. Breakpoints can still be placed in the code and will be triggered when the page is served.