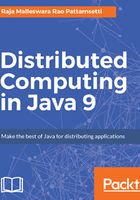
RMI for distributed computing
Remote objects are similar to local objects when it comes to method invocation and returning results from methods. A remote object can be typecasted to any of the remote interfaces supported by its implementation, and the instanceof operator can be used to verify the type of remote or local object.
Although the aforementioned similarities exist between remote and local objects, they behave differently. While the clients of remote objects can only interact with remote interfaces, not with implementation classes, the clients of local objects can interact with both the interfaces and implementation classes. RMI is passed by a value, whereas a local method invocation can be passed by a reference. In this context, the mandatory remote exceptions must be defined in a local system.
The essential steps that need to be followed to develop a distributed application with RMI are as follows:
- Design and implement a component that should not only be involved in the distributed application, but also the local components.
- Ensure that the components that participate in the RMI calls are accessible across networks.
- Establish a network connection between applications that need to interact using the RMI.
In the preceding list, the most important step, which needs to be taken carefully, is defining the right architecture in the application with clear distinction between the components that act as Java objects available on a local JVM and ones that are remotely accessible. Let's review the implementation steps in detail:
- Remote interface definition: The purpose of defining a remote interface is to declare the methods that should be available for invocation by a remote client. Programming the interface instead of programming the component implementation is an essential design principle adopted by all modern Java frameworks, including Spring. In the same pattern, the definition of a remote interface takes importance in RMI design as well.
- Remote object implementation: Java allows a class to implement more than one interface at a time. This helps remote objects implement one or more remote interfaces. The remote object class may have to implement other local interfaces and methods that it is responsible for. Avoid adding complexity to this scenario, in terms of how the arguments or return parameter values of such component methods should be written.
- Remote client implementation: Client objects that interact with remote server objects can be written once the remote interfaces are carefully defined even after the remote objects are deployed.
Now let's consider a simple example and how we can implement it to stimulate interaction between multiple JVMs using the Java RMI.