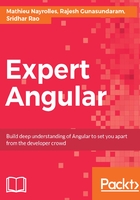
上QQ阅读APP看书,第一时间看更新
Namespaces
We can create namespaces in TypeScript using the namespace keyword as follows. All the classes defined under namespace will be scoped under this namespace and will not be attached to the global scope:
namespace Inventory { class Product { constructor (public name: string, public quantity: number) { } } // product is accessible var p = new Product('mobile', 101); } // Product class is not accessible outside namespace var p = new Inventory.Product('mobile', 101);
To make the Product class available for access outside the namespace, we need to add an export keyword when defining the Product class, as follows:
module Inventory { export class Product { constructor (public name: string, public quantity: number) { } } } // Product class is now accessible outside namespace var p = new Inventory.Product('mobile', 101);
We can also share namespaces across files by adding a reference statement at the beginning of the code in the referring files, as follows:
/// <reference path="Inventory.ts" />