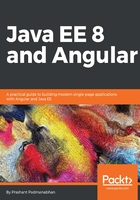
RequestContext Activation
When we talk about context in Java EE, we are presented with various options, such as Application, Request, Session, and Conversation context, but not everything makes sense in an SE environment. While the Application context can be present in SE, which starts with the container boot and lives till the container is shutdown, there isn't support for any other context. The only additional context which is supported in CDI 2.0 is the request context. There are two approaches to obtain the request context it can be framework managed or it can be programmatically managed by the developer.
For programmatic access, you need to inject the RequestContextController instance in your class and then use its activate() and deactivate() methods to work with the request context in the current thread. Calling activate() within a thread will activate the request context if not already active. Similarly, deactivate() will stop the active request context if it exists, but may throw a ContextNotActiveException otherwise. Here’s the API-based method of request context control:
@ApplicationScoped
public class Task {
@Inject
private RequestContextController requestContextController;
public void doWorkInRequest(String data) {
boolean activated = requestContextController.activate();
//some work here
if(activated) requestContextController.deactivate();
}
}
For the container to automatically start a request context, an interceptor binding annotation of @ActivateRequestContext can be used, which is bound to the method execution. Here's an example of this in action:
@ApplicationScoped
public class Task {
@ActivateRequestContext
public void doWorkInRequest(String data) {
//some work here, with request context activated
}
}
With the preceding code, if you had a RequestScoped bean injected into the Task class, then the same would be created for each invocation of the doWorkInRequest method.
In a Java SE application, the notion of request may not sound right, as Java EE developers would typically talk of requests as HTTP requests. But if you consider some GUI applications where a button is clicked, then you can relate this to a click event which is fired as part of the button click request.