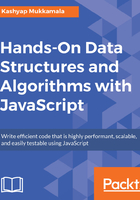
Building a basic web worker
Once we have the app created and instantiated, we will create the worker.js file first using the following commands from the root of your app:
cd src/app
mkdir utils
touch worker.js
This will generate the utils folder and the worker.js file in it.
Note the following two things here:
- It is a simple JS file and not a TypeScript file, even though the entire application is in TypeScript
- It is called worker.js, which means that we will be creating a web worker for the parsing and evaluation that we are about to perform
Web workers are used to simulate the concept of multithreading in JavaScript, which is usually not the case. Also, since this thread runs in isolation, there is no way for us to provide dependencies to that. This works out very well for us because our main app is only going to accept the user's input and provide it to the worker on every key stroke while it's the responsibility of the worker to evaluate this expression and return the result or the error if necessary.
Since this is an external file and not a standard Angular file, we will have to load it up as an external script so that our application can use it subsequently. To do so, open your .angular-cli.json file and update the scripts option to look as follows:
...
"scripts": [
"app/utils/worker.js"
],
...
Now, we will be able to use the injected worker, as follows:
this.worker = new Worker('scripts.bundle.js');
First, we will add the necessary changes to the app.component.ts file so that it can interact with worker.js as needed.