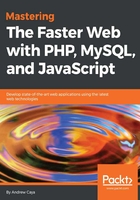
Practical prerequisites
In order to run the source code included in this book, we recommend that you start by installing Docker on your computer (https://docs.docker.com/engine/installation/). Docker is a software container platform that allows you to easily connect to your computer's devices in an isolated and sophisticated chroot-like environment. Unlike virtual machines, containers do not come bundled with full operating systems, but rather come with the required binaries in order to run some software. You can install Docker on Windows, Mac, or Linux. It should be noted, however, that some features, like full-featured networking, are still not available when running Docker on macOS (https://docs.docker.com/docker-for-mac/networking/#known-limitations-use-cases-and-workarounds).
The main Docker image that we will be using throughout this book is Linux for PHP 8.1 (https://linuxforphp.net/) with a non-thread safe version of PHP 7.1.16 and MariaDB (MySQL) 10.2.8 (asclinux/linuxforphp-8.1:7.1.16-nts). Once Docker is installed on your computer, please run the following commands in a bash-like Terminal in order to get a copy of the book's code examples and start the appropriate Docker container:
# git clone https://github.com/andrewscaya/fasterweb # cd fasterweb # docker run --rm -it \ -v ${PWD}/:/srv/fasterweb \ -p 8181:80 \ asclinux/linuxforphp-8.1:7.1.16-nts \ /bin/bash
After running these commands, you should get the following command prompt:

The Linux for PHP container’s command line interface (CLI)
Note to Windows users: please make sure to replace the '${PWD}' portion of the shared volumes option in the previous Docker command with the full path to your working directory (ex. '/c/Users/fasterweb'), because you will not be able to start the container otherwise. Also, you should make sure that volume sharing is enabled in your Docker settings. Moreover, if you are running Docker on Windows 7 or 8, you will only be able to access the container at the address http://192.168.99.100:8181 and not at 'localhost:8181'.
All the code examples given in this book can be found, within the code repository, in a folder named according to the chapter's number. Thus, it is expected that you change your working directory at the beginning of each chapter in order to run the code examples given within. Thus, for this chapter, you are expected to enter, on the container's CLI, the following commands:
# mv /srv/www /srv/www.OLD
# ln -s /srv/fasterweb/chapter_1 /srv/www
And, for the next chapter, you are expected to enter these commands:
# rm /srv/www
# ln -s /srv/fasterweb/chapter_2 /srv/www
And, so on for the following chapters.
Also, if you prefer using multithreading technologies while optimizing your code, you can do so by running the thread-safe version of Linux for PHP (asclinux/linuxforphp-8.1:7.0.29-zts).
If you prefer running the container in detached mode (-d switch), please do so. This will allow you to docker exec many command shells against the same container while keeping it up and running at all times independently of whether you have a running Terminal or not.
Moreover, you should docker commit any changes you made to the container and create new images of it so that you can docker run it at a later time. If you are not familiar with the Docker command line and its run command, please find the documentation at the following address: https://docs.docker.com/engine/reference/run/.
Finally, many excellent books and videos on Docker have been published by Packt Publishing and I highly recommend that you read them in order to master this fine tool.
Now, enter the following commands in order to start all the services that will be needed throughout this book and to create a test script that will allow you to make sure everything is working as expected:
# cd /srv/www
# /etc/init.d/mysql start # /etc/init.d/php-fpm start # /etc/init.d/httpd start # touch /srv/www/index.php # echo -e "<?php phpinfo();" > /srv/www/index.php
Once you are done running these commands, you should point your favorite browser to http://localhost:8181/ and see the following result:

The phpinfo page
If you do not see this page, please try to troubleshoot your Docker installation.
Moreover, please note that, if you do not docker commit your changes and prefer to use an original Linux for PHP base image whenever you wish to start working with a code example contained in this book, the previous commands will have to be repeated each and every time.
We are now ready to benchmark our server.