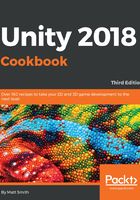
上QQ阅读APP看书,第一时间看更新
How to do it...
To wait for audio to finish playing before destroying its parent GameObject, follow these steps:
- Create a new Unity 2D project and import the sound clip file.
- Create a GameObject in the scene containing an AudioSource component linked to the AudioClip engineSound. This can be done in a single step by dragging the music clip from the Project panel into either the Hierarchy or Scene panels. Rename this the AudioObject GameObject.
- Uncheck the Play Awake property for the AudioSource component of the GameObject engineSound – so this sound does not begin playing as soon as the scene is loaded.
- Create a C# script class, AudioDestructBehaviour, in a new folder, _Scripts, containing the following code, and add an instance as a scripted component to the AudioObject GameObject:
using UnityEngine; using UnityEngine; public class AudioDestructBehaviour : MonoBehaviour { private AudioSource audioSource; void Awake() { audioSource = GetComponent<AudioSource>(); } private void Update() { if( !audioSource.isPlaying ) Destroy(gameObject); } }
- In the Inspector panel, disable (uncheck) the AudioDestructBehaviour scripted component of AudioObject (when needed, it will be re-enabled via C# code):

- Create a C# script class, ButtonActions, in the _Scripts folder, containing the following code, and add an instance as a scripted component to the Main Camera GameObject:
using UnityEngine; public class ButtonActions : MonoBehaviour { public AudioSource audioSource; public AudioDestructBehaviour audioDestructScriptedObject; public void ACTION_PlaySound() { if( !audioSource.isPlaying ) audioSource.Play(); } public void ACTION_DestroyAfterSoundStops(){ audioDestructScriptedObject.enabled = true; } }
- With Main Camera selected in the Hierarchy panel, drag AudioObject into
the Inspector panel for the public Audio Source variable. - With Main Camera selected in the Hierarchy panel, drag AudioObject into
the Inspector panel for the public Audio Destruct Scripted Object variable. - Create a UI button named Button-play-sound, changing its text to Play Sound. Position the button in the center of the screen by setting its Rect Transform property to middle-center.
- With Button-play-sound selected in the Hierarchy panel, create a new on-click event-handler, dragging the Main Camera into the Object slot, and selecting the ACTION_PlaySound() function.
- Create a second UI button named Button-destroy-when-finished-playing, changing its text to Destroy When Sound Finished. Position the button in the center of the screen (just below the other button) by setting its Rect Transform property to middle-center and then drag the button down a little.
- With Button-destroy-when-finished-playing selected in the Hierarchy panel, create a new on-click event-handler, dragging the Main Camera into the Object slot, and selecting the ACTION_ DestroyAfterSoundStops() function.
- Run the scene. Clicking the Play Sound button will play the engine sound each time. However, once the Destroy When Sound Finished button has been clicked, as soon as the engineSound finished playing, you'll see the AudioObject GameObject disappear from the Hierarchy panel, since the GameObject has destroyed itself.