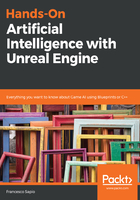
Creating a NavArea class in C++
It's easy to create a NavArea class in C++ as well. First of all, you need to create a new C++ class that inherits from the NavArea class, as shown in the following screenshot:

By convention, the name should start with "NavArea_". Therefore, you can rename it NavArea_Desert (just to vary which kind of terrain the AI can face, since we created a Jungle previously) and place it in "Chapter3/Navigation":

Once you have created the class, you just need to assign the parameters in the constructor. For your convenience, here is the class definition in which we declare a simple constructor:
#include "CoreMinimal.h"
#include "NavAreas/NavArea.h"
#include "NavArea_Desert.generated.h"
/**
*
*/
UCLASS()
class UNREALAIBOOK_API UNavArea_Desert : public UNavArea
{
GENERATED_BODY()
UNavArea_Desert();
};
Then, in the implementation of the constructor, we can assign the different parameters. For instance, we can have a high cost for entering and a higher cost for traversing (with respect to the Default or the Jungle). Furthermore, we can set the color to Yellow so that we remember that it is a desert area:
#include "NavArea_Desert.h"
UNavArea_Desert::UNavArea_Desert()
{
DefaultCost = 1.5f;
FixedAreaEnteringCost = 3.f;
DrawColor = FColor::Yellow;
}
Once you have created the class, you can set it as part of the Nav Modifier Volume, as shown in the following screenshot:

As a result, you will be able to see your custom area in the Nav Mesh (in this case, with a Yellow Color):
