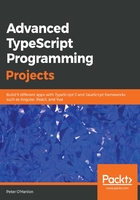
Coping with a variable number of parameters using REST
The final thing we need to look at with regard to REST is the idea of functions having REST parameters. These aren't the same as REST properties but the syntax is so similar that we should find it easy to pick up. The problem that REST parameters solves is to cope with a variable number of parameters being passed into a function. The way to identify a REST parameter in a function is that it is preceded by the ellipsis and that it is typed as an array.
In this example, we are going to log out a header followed by a variable number of instruments:
function PrintInstruments(log : string, ...instruments : string[]) : void {
console.log(log);
instruments.forEach(instrument => {
console.log(instrument);
});
}
PrintInstruments('Music Shop Inventory', 'Guitar', 'Drums', 'Clarinet', 'Clavinova');
As the REST parameter is an array, this gives us access to array functions, which means that we can perform actions such as forEach from it directly. Importantly, REST parameters are different from the arguments object inside a JavaScript function because they start at the values that have not been named in the parameters list, whereas the arguments object contains a list of all of the arguments.
As REST parameters were not available in ES5, TypeScript does the work necessary to provide JavaScript that simulates the REST parameter. First, we will see what this looks like when compiled as ES5, as follows:
function PrintInstruments(log) {
var instruments = [];
// As our rest parameter starts at the 1st position in the list of
// arguments,
// our index starts at 1.
for (var _i = 1; _i < arguments.length; _i++) {
instruments[_i - 1] = arguments[_i];
}
console.log(log);
instruments.forEach(function (instrument) {
console.log(instrument);
});
}
When we look at the JavaScript produced from an ES2015 compilation (you will need to change the entry for target to ES2015 in the tsconfig.json file), we see that it looks exactly the same as our TypeScript code:
function PrintInstruments(log, ...instruments) {
console.log(log);
instruments.forEach(instrument => {
console.log(instrument);
});
}
At this point, I cannot stress enough how important it is to take a look at the JavaScript that is being produced. TypeScript is very good at hiding complexity from us, but we really should be familiar with what is being produced. I find it a great way to understand what is going on under the covers, where possible, to compile using different versions of the ECMAScript standard and see what code is being produced.